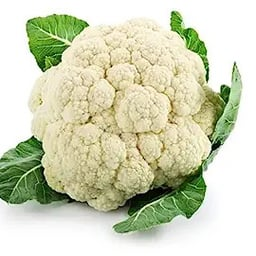

The NewPipe team deserve a Nobel Prize. It might look like an unimportant video player, but it’s one of the last bastion of resistance against he Google monopoly and monoculture, the total invasion of advertisement in every corner of our lives, the privacy violation that always come with Google, and the general enshittification of the internet.
Thank goodness for NewPipe…
My “everyday carry” isn’t a USB stick, but it can act as one - and much much more: I always have my trusty Flipper Zero with me, and the image I carry in the mass storage emulator is the Linux Mint installer, with extra space in the image to store small files.
To be honest, the Flipper Zero’s mass storage emulator turns it into the slowest USB stick you never saw. But in a pinch, it’s there and it’s usable. I use my Flipper for a variety of other things all the time - including, with my laptop, as a presentation remote and secondary mouse - and I almost never need a USB flash drive. So slow though it is, it’s enough for when I do need one.